The Tau Collections
Contents
The Tau Collections¶
Taus are complex jet-like objects that are reconstructed and calibrated with their own algorithms. They are a lot like jets, from a data model point-of-view.
import matplotlib.pyplot as plt
from config import ds_ztautau as ds
import awkward as ak
from func_adl_servicex_xaodr21 import calib_tools
from helpers import match_objects
By default we fetch tau-jets from the Tight
working point (you can change the working point by passing the working_point
argument).
taus = (ds
.Select(lambda e: e.TauJets())
.Select(lambda ts: {
'pt': [t.pt()/1000.0 for t in ts],
'eta': [t.eta() for t in ts],
'phi': [t.phi() for t in ts],
})
.AsAwkwardArray()
.value())
plt.hist(ak.flatten(taus.pt), bins=100, range=(0, 100))
plt.xlabel('Tau $p_T$ [GeV]')
plt.ylabel('Number of Taus')
_ = plt.title('Tau $p_T$ distribution for $Z\\rightarrow \\tau \\tau$ events')
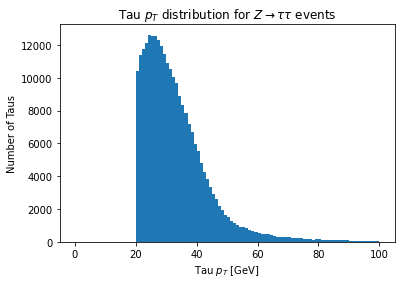
Calibration¶
By default the taus we pulled from above are calibrated, and the best central value for the tau collection you request is returned. We use the standard approach to get both uncalibrated versions of the collection and systematic error variations.
To grab the raw jets (without calibration) we just set the uncalibrated_collection
parameter to TauJets
(there is very little reason one will do this normally):
raw_taus = (ds
.Select(lambda e: e.TauJets(uncalibrated_collection="TauJets"))
.Select(lambda ts: {
'pt': [t.pt()/1000.0 for t in ts],
'eta': [t.eta() for t in ts],
'phi': [t.phi() for t in ts],
})
.AsAwkwardArray()
.value())
The number of taus and the number of calibrated taus are quite different, so we’ll need to match them in \(\eta\) and \(\phi\):
raw_taus_matched = match_objects(taus, raw_taus)
plt.hist(ak.flatten(taus.pt-raw_taus_matched.pt), bins=100, range=(-10, 10))
plt.xlabel('$\Delta p_T$ for calibrated taus matched to their raw taus [GeV]')
plt.ylabel('Number of Taus')
_ = plt.title('The effect of tau calibration on tau $p_T$ in $Z\\rightarrow \\tau\\tau$ events')
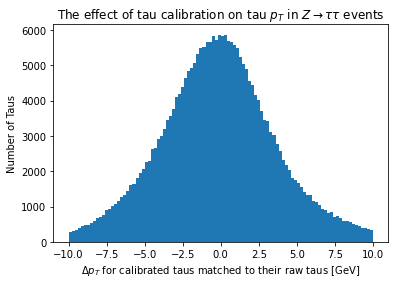
If we instead want a particular systematic error, we need only name that error to get it back. Knowing what the names of the systematic errors, however, is not something that can be programmatically determined ahead of time. See the further information section at the end of this chapter to links to the ATLAS jet calibration info twiki.
sys_taus = (calib_tools.query_sys_error(ds, 'TAUS_TRUEHADTAU_SME_TES_DETECTOR__1up')
.Select(lambda e: e.TauJets())
.Select(lambda ts: {
'pt': [t.pt()/1000.0 for t in ts],
'eta': [t.eta() for t in ts],
'phi': [t.phi() for t in ts],
})
.AsAwkwardArray()
.value())
sys_taus_matched = match_objects(taus, sys_taus)
plt.hist(ak.flatten(taus.pt-sys_taus_matched.pt), bins=100, range=(-5, 5))
plt.xlabel(r'$\Delta p_T$ for calibrated taus matched to their $\phi-\eta$ raw taus [GeV]')
plt.ylabel('Number of Taus')
plt.yscale('log')
_ = plt.title(r'The effect of a tau calibration sys error on tau $p_T$ in $Z\rightarrow \tau\tau$ events')
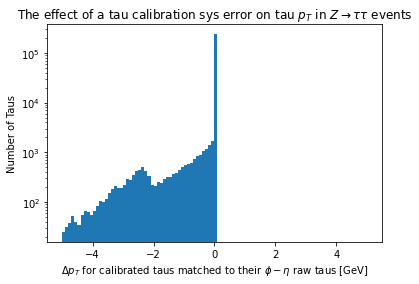
The Datamodel¶
The data model when this documentation was last built was:
from func_adl_servicex_xaodr21.xAOD.taujet_v3 import TauJet_v3
help(TauJet_v3)
Help on class TauJet_v3 in module func_adl_servicex_xaodr21.xAOD.taujet_v3:
class TauJet_v3(builtins.object)
| A class
|
| Methods defined here:
|
| ROIWord(self) -> 'int'
| A method
|
| allTauTrackLinks(self) -> 'func_adl_servicex_xaodr21.vector_elementlink_datavector_xaod_tautrack_v1___.vector_ElementLink_DataVector_xAOD_TauTrack_v1___'
| A method
|
| allTauTrackLinksNonConst(self) -> 'func_adl_servicex_xaodr21.vector_elementlink_datavector_xaod_tautrack_v1___.vector_ElementLink_DataVector_xAOD_TauTrack_v1___'
| A method
|
| charge(self) -> 'float'
| A method
|
| chargedPFO(self, i: 'int') -> 'func_adl_servicex_xaodr21.xAOD.pfo_v1.PFO_v1'
| A method
|
| chargedPFOLinks(self) -> 'func_adl_servicex_xaodr21.vector_elementlink_datavector_xaod_pfo_v1___.vector_ElementLink_DataVector_xAOD_PFO_v1___'
| A method
|
| clearDecorations(self) -> 'bool'
| A method
|
| cluster(self, i: 'int') -> 'func_adl_servicex_xaodr21.xAOD.iparticle.IParticle'
| A method
|
| clusterLinks(self) -> 'func_adl_servicex_xaodr21.vector_elementlink_datavector_xaod_iparticle___.vector_ElementLink_DataVector_xAOD_IParticle___'
| A method
|
| e(self) -> 'float'
| A method
|
| eta(self) -> 'float'
| A method
|
| etaDetectorAxis(self) -> 'float'
| A method
|
| etaFinalCalib(self) -> 'float'
| A method
|
| etaIntermediateAxis(self) -> 'float'
| A method
|
| etaJetSeed(self) -> 'float'
| A method
|
| etaPanTauCellBased(self) -> 'float'
| A method
|
| etaPanTauCellBasedProto(self) -> 'float'
| A method
|
| etaTauEnergyScale(self) -> 'float'
| A method
|
| etaTauEtaCalib(self) -> 'float'
| A method
|
| etaTrigCaloOnly(self) -> 'float'
| A method
|
| hadronicPFO(self, i: 'int') -> 'func_adl_servicex_xaodr21.xAOD.pfo_v1.PFO_v1'
| A method
|
| hadronicPFOLinks(self) -> 'func_adl_servicex_xaodr21.vector_elementlink_datavector_xaod_pfo_v1___.vector_ElementLink_DataVector_xAOD_PFO_v1___'
| A method
|
| hasNonConstStore(self) -> 'bool'
| A method
|
| hasStore(self) -> 'bool'
| A method
|
| index(self) -> 'int'
| A method
|
| jet(self) -> 'func_adl_servicex_xaodr21.xAOD.jet_v1.Jet_v1'
| A method
|
| jetLink(self) -> 'func_adl_servicex_xaodr21.elementlink_datavector_xaod_jet_v1__.ElementLink_DataVector_xAOD_Jet_v1__'
| A method
|
| m(self) -> 'float'
| A method
|
| mDetectorAxis(self) -> 'float'
| A method
|
| mFinalCalib(self) -> 'float'
| A method
|
| mIntermediateAxis(self) -> 'float'
| A method
|
| mJetSeed(self) -> 'float'
| A method
|
| mPanTauCellBased(self) -> 'float'
| A method
|
| mPanTauCellBasedProto(self) -> 'float'
| A method
|
| mTauEnergyScale(self) -> 'float'
| A method
|
| mTauEtaCalib(self) -> 'float'
| A method
|
| mTrigCaloOnly(self) -> 'float'
| A method
|
| nAllTracks(self) -> 'int'
| A method
|
| nChargedPFOs(self) -> 'int'
| A method
|
| nClusters(self) -> 'int'
| A method
|
| nHadronicPFOs(self) -> 'int'
| A method
|
| nNeutralPFOs(self) -> 'int'
| A method
|
| nPi0PFOs(self) -> 'int'
| A method
|
| nPi0s(self) -> 'int'
| A method
|
| nProtoChargedPFOs(self) -> 'int'
| A method
|
| nProtoNeutralPFOs(self) -> 'int'
| A method
|
| nProtoPi0PFOs(self) -> 'int'
| A method
|
| nShotPFOs(self) -> 'int'
| A method
|
| nTracksCharged(self) -> 'int'
| A method
|
| nTracksIsolation(self) -> 'int'
| A method
|
| nTracksWithMask(self, classification: 'int') -> 'int'
| A method
|
| neutralPFO(self, i: 'int') -> 'func_adl_servicex_xaodr21.xAOD.pfo_v1.PFO_v1'
| A method
|
| neutralPFOLinks(self) -> 'func_adl_servicex_xaodr21.vector_elementlink_datavector_xaod_pfo_v1___.vector_ElementLink_DataVector_xAOD_PFO_v1___'
| A method
|
| p4(self) -> 'func_adl_servicex_xaodr21.tlorentzvector.TLorentzVector'
| A method
|
| phi(self) -> 'float'
| A method
|
| phiDetectorAxis(self) -> 'float'
| A method
|
| phiFinalCalib(self) -> 'float'
| A method
|
| phiIntermediateAxis(self) -> 'float'
| A method
|
| phiJetSeed(self) -> 'float'
| A method
|
| phiPanTauCellBased(self) -> 'float'
| A method
|
| phiPanTauCellBasedProto(self) -> 'float'
| A method
|
| phiTauEnergyScale(self) -> 'float'
| A method
|
| phiTauEtaCalib(self) -> 'float'
| A method
|
| phiTrigCaloOnly(self) -> 'float'
| A method
|
| pi0(self, i: 'int') -> 'func_adl_servicex_xaodr21.xAOD.iparticle.IParticle'
| A method
|
| pi0ConeDR(self) -> 'float'
| A method
|
| pi0Links(self) -> 'func_adl_servicex_xaodr21.vector_elementlink_datavector_xaod_iparticle___.vector_ElementLink_DataVector_xAOD_IParticle___'
| A method
|
| pi0PFO(self, i: 'int') -> 'func_adl_servicex_xaodr21.xAOD.pfo_v1.PFO_v1'
| A method
|
| pi0PFOLinks(self) -> 'func_adl_servicex_xaodr21.vector_elementlink_datavector_xaod_pfo_v1___.vector_ElementLink_DataVector_xAOD_PFO_v1___'
| A method
|
| protoChargedPFO(self, i: 'int') -> 'func_adl_servicex_xaodr21.xAOD.pfo_v1.PFO_v1'
| A method
|
| protoChargedPFOLinks(self) -> 'func_adl_servicex_xaodr21.vector_elementlink_datavector_xaod_pfo_v1___.vector_ElementLink_DataVector_xAOD_PFO_v1___'
| A method
|
| protoNeutralPFO(self, i: 'int') -> 'func_adl_servicex_xaodr21.xAOD.pfo_v1.PFO_v1'
| A method
|
| protoNeutralPFOLinks(self) -> 'func_adl_servicex_xaodr21.vector_elementlink_datavector_xaod_pfo_v1___.vector_ElementLink_DataVector_xAOD_PFO_v1___'
| A method
|
| protoPi0PFO(self, i: 'int') -> 'func_adl_servicex_xaodr21.xAOD.pfo_v1.PFO_v1'
| A method
|
| protoPi0PFOLinks(self) -> 'func_adl_servicex_xaodr21.vector_elementlink_datavector_xaod_pfo_v1___.vector_ElementLink_DataVector_xAOD_PFO_v1___'
| A method
|
| pt(self) -> 'float'
| A method
|
| ptDetectorAxis(self) -> 'float'
| A method
|
| ptFinalCalib(self) -> 'float'
| A method
|
| ptIntermediateAxis(self) -> 'float'
| A method
|
| ptJetSeed(self) -> 'float'
| A method
|
| ptPanTauCellBased(self) -> 'float'
| A method
|
| ptPanTauCellBasedProto(self) -> 'float'
| A method
|
| ptTauEnergyScale(self) -> 'float'
| A method
|
| ptTauEtaCalib(self) -> 'float'
| A method
|
| ptTrigCaloOnly(self) -> 'float'
| A method
|
| rapidity(self) -> 'float'
| A method
|
| secondaryVertex(self) -> 'func_adl_servicex_xaodr21.xAOD.vertex_v1.Vertex_v1'
| A method
|
| secondaryVertexLink(self) -> 'func_adl_servicex_xaodr21.elementlink_datavector_xaod_vertex_v1__.ElementLink_DataVector_xAOD_Vertex_v1__'
| A method
|
| shotPFO(self, i: 'int') -> 'func_adl_servicex_xaodr21.xAOD.pfo_v1.PFO_v1'
| A method
|
| shotPFOLinks(self) -> 'func_adl_servicex_xaodr21.vector_elementlink_datavector_xaod_pfo_v1___.vector_ElementLink_DataVector_xAOD_PFO_v1___'
| A method
|
| tauTrackLinksWithMask(self, noname_arg: 'int') -> 'func_adl_servicex_xaodr21.vector_elementlink_datavector_xaod_tautrack_v1___.vector_ElementLink_DataVector_xAOD_TauTrack_v1___'
| A method
|
| trackFilterProngs(self) -> 'int'
| A method
|
| trackFilterQuality(self) -> 'int'
| A method
|
| trackWithMask(self, i: 'int', mask: 'int', container_index: 'int') -> 'func_adl_servicex_xaodr21.xAOD.tautrack_v1.TauTrack_v1'
| A method
|
| usingPrivateStore(self) -> 'bool'
| A method
|
| usingStandaloneStore(self) -> 'bool'
| A method
|
| vertex(self) -> 'func_adl_servicex_xaodr21.xAOD.vertex_v1.Vertex_v1'
| A method
|
| vertexLink(self) -> 'func_adl_servicex_xaodr21.elementlink_datavector_xaod_vertex_v1__.ElementLink_DataVector_xAOD_Vertex_v1__'
| A method
|
| ----------------------------------------------------------------------
| Readonly properties defined here:
|
| auxdataConst
| A method
|
| isAvailable
| A method
|
| ----------------------------------------------------------------------
| Data descriptors defined here:
|
| __dict__
| dictionary for instance variables (if defined)
|
| __weakref__
| list of weak references to the object (if defined)
Further Information¶
The
xAOD::TauJet_v3
C++ header file with all the inline documentation.The Tau Recommendation Pages for R21 on the ATLAS TWiki