The Track Collection
Contents
The Track Collection¶
This is the first collection of objects in the xAOD event model that does not require any CP calibration code to be executed before accessing it. Tracks in ATLAS are straight forward - you apply the cuts on your own (or they are done by a derivation). As a result, tracks are simple objects.
Note that tracks are hefty objects - and as a result they are often targets of slimming and thinning. The latter is especially problematic as a method on the Track
object will fail with an AUX error - because the data backing it is not actually present in the file.
import matplotlib.pyplot as plt
from config import ds_zee as ds
Fetch all the inner detector tracks and plot their \(p_T\) and the radius of the first hit on the tracks. Note that unlike the calibrated objects, you must supply the name of the track collection to the TrackParticles
call. There is no default.
tracks = (ds
.SelectMany(lambda e: e.TrackParticles("InDetTrackParticles"))
.Select(lambda t:
{
"pt": t.pt() / 1000.0,
})
.AsAwkwardArray()
.value())
plt.hist(tracks.pt, bins=100, range=(0, 10))
plt.xlabel('Track $p_T$ [GeV]')
plt.ylabel('Number of tracks')
_ = plt.title('Track $p_T$ distribution for $Z\\rightarrow ee$ events')
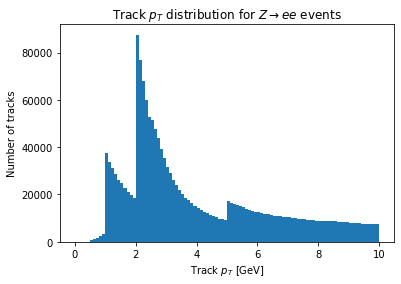
The Datamodel¶
The data model when this documentation was last built was:
from func_adl_servicex_xaodr21.xAOD.trackparticle_v1 import TrackParticle_v1
help(TrackParticle_v1)
Help on class TrackParticle_v1 in module func_adl_servicex_xaodr21.xAOD.trackparticle_v1:
class TrackParticle_v1(builtins.object)
| A class
|
| Methods defined here:
|
| beamlineTiltX(self) -> 'float'
| A method
|
| beamlineTiltY(self) -> 'float'
| A method
|
| charge(self) -> 'float'
| A method
|
| chiSquared(self) -> 'float'
| A method
|
| clearDecorations(self) -> 'bool'
| A method
|
| d0(self) -> 'float'
| A method
|
| definingParametersCovMatrixVec(self) -> 'func_adl_servicex_xaodr21.vector_float_.vector_float_'
| A method
|
| e(self) -> 'float'
| A method
|
| eta(self) -> 'float'
| A method
|
| hasNonConstStore(self) -> 'bool'
| A method
|
| hasStore(self) -> 'bool'
| A method
|
| hitPattern(self) -> 'int'
| A method
|
| index(self) -> 'int'
| A method
|
| m(self) -> 'float'
| A method
|
| numberDoF(self) -> 'float'
| A method
|
| numberOfParameters(self) -> 'int'
| A method
|
| p4(self) -> 'func_adl_servicex_xaodr21.tlorentzvector.TLorentzVector'
| A method
|
| parameterPX(self, index: 'int') -> 'float'
| A method
|
| parameterPY(self, index: 'int') -> 'float'
| A method
|
| parameterPZ(self, index: 'int') -> 'float'
| A method
|
| parameterX(self, index: 'int') -> 'float'
| A method
|
| parameterY(self, index: 'int') -> 'float'
| A method
|
| parameterZ(self, index: 'int') -> 'float'
| A method
|
| phi(self) -> 'float'
| A method
|
| phi0(self) -> 'float'
| A method
|
| pt(self) -> 'float'
| A method
|
| qOverP(self) -> 'float'
| A method
|
| radiusOfFirstHit(self) -> 'float'
| A method
|
| rapidity(self) -> 'float'
| A method
|
| theta(self) -> 'float'
| A method
|
| usingPrivateStore(self) -> 'bool'
| A method
|
| usingStandaloneStore(self) -> 'bool'
| A method
|
| vertex(self) -> 'func_adl_servicex_xaodr21.xAOD.vertex_v1.Vertex_v1'
| A method
|
| vertexLink(self) -> 'func_adl_servicex_xaodr21.elementlink_datavector_xaod_vertex_v1__.ElementLink_DataVector_xAOD_Vertex_v1__'
| A method
|
| vx(self) -> 'float'
| A method
|
| vy(self) -> 'float'
| A method
|
| vz(self) -> 'float'
| A method
|
| z0(self) -> 'float'
| A method
|
| ----------------------------------------------------------------------
| Readonly properties defined here:
|
| auxdataConst
| A method
|
| isAvailable
| A method
|
| ----------------------------------------------------------------------
| Data descriptors defined here:
|
| __dict__
| dictionary for instance variables (if defined)
|
| __weakref__
| list of weak references to the object (if defined)
Further Information¶
The
xAOD::TrackParticle_v1
C++ header file with all the inline documentation.The Tracking CP group R21 recommendation pages on the ATLAS TWiki